“ 本文描述了如何在Winform使用DataGridView表格控件实现实现数据的添加,编辑、删除、分页显示功能。”
Winform
WinForm(Windows Forms)是 Microsoft .NET Framework 提供的一个图形用户界面(GUI)框架,使用Winform能简单快速开发桌面工具程序。
DataGridView
DataGridView 是 Windows Forms 中功能强大且灵活的表格控件,用于显示和编辑表格数据。它是 .NET Framework 中替代旧版 DataGrid 控件的主要选择。
案例代码使用Visual Studio2022编程软件,其提供了对WinForm的完整支持,包括可视化设计器和丰富的工具箱,非常适合新人快速上手。要实现表格中添加按钮功能,需要使用DataGridView 控件中的列类型 DataGridViewButtonColumn ,其专门用于在表格中显示按钮。它的主要作用是为用户提供交互式操作(如点击按钮触发特定功能),通过CellContentClick事件判断按钮点击项,实现“编辑”、“删除”操作。
DataGridView的基本特性
支持多种数据源绑定(DataTable、List、数组等)
提供丰富的单元格格式设置选项
支持排序、过滤和分组功能允许自定义列类型(文本框、复选框、组合框等)
提供行和列的添加、删除、编辑功能
运行效果
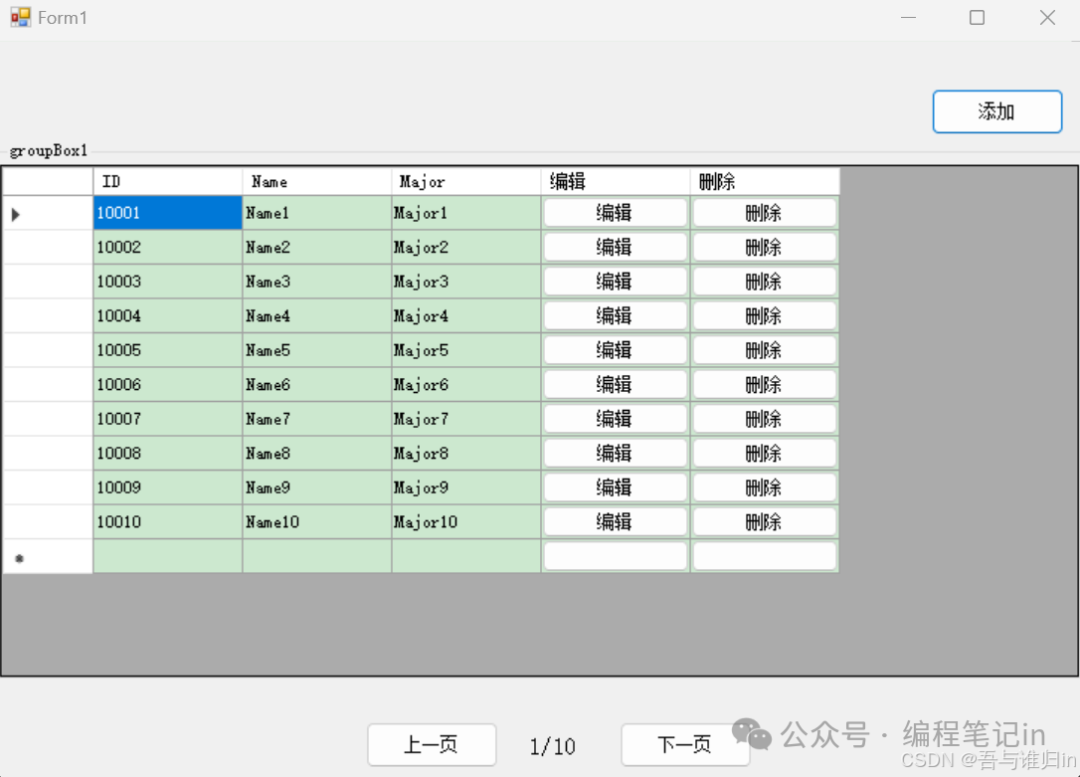
代码
1、窗体代码
public partial class Form1 : Form
{
private BindingSource bindingSource = new BindingSource();
private List<Student> students = new List<Student>();
private int currentPage = 0;
private int pageSize = 10;
public Form1()
{
InitializeComponent();
CenterToParent();
CenterToScreen();
LoadData();
bindingSource.DataSource = GetPagedData(0, pageSize);
dataGridView1.DataSource = bindingSource;
DataGridViewButtonColumn editButtonColumn = new DataGridViewButtonColumn();
editButtonColumn.Name = "Edit";
editButtonColumn.HeaderText = "编辑";
editButtonColumn.Text = "编辑";
editButtonColumn.UseColumnTextForButtonValue = true;
dataGridView1.Columns.Add(editButtonColumn);
DataGridViewButtonColumn deleteButtonColumn = new DataGridViewButtonColumn();
deleteButtonColumn.Name = "Delete";
deleteButtonColumn.HeaderText = "删除";
deleteButtonColumn.Text = "删除";
deleteButtonColumn.UseColumnTextForButtonValue = true;
dataGridView1.Columns.Add(deleteButtonColumn);
Label_CurrentPageShow.Text = $"{currentPage+1}/{students.Count / 10}";
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void LoadData()
{
for (int i = 1; i <= 100; i++)
{
string id = (10000+i).ToString();
students.Add(new Student { ID = id, Name = "Name" + i, Major = "Major" + i });
}
}
private List<Student> GetPagedData(int pageIndex, int pageSize)
{
return students.Skip(pageIndex * pageSize).Take(pageSize).ToList();
}
private void btnPrevious_Click(object sender, EventArgs e)
{
if (currentPage > 0)
{
currentPage--;
bindingSource.DataSource = GetPagedData(currentPage, pageSize);
ShowPageNumber();
}
}
private void btnNext_Click(object sender, EventArgs e)
{
if ((currentPage + 1) * pageSize < students.Count)
{
currentPage++;
bindingSource.DataSource = GetPagedData(currentPage, pageSize);
ShowPageNumber();
}
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex >= 0)
{
var student = (Student)bindingSource[e.RowIndex];
if (e.ColumnIndex == dataGridView1.Columns["Edit"].Index)
{
EditStudent(student);
}
else if (e.ColumnIndex == dataGridView1.Columns["Delete"].Index)
{
DeleteStudent(student);
}
}
}
private void EditStudent(Student student)
{
MessageBox.Show($"编辑: {student.Name}");
}
private void DeleteStudent(Student student)
{
if (MessageBox.Show($"删除用户:{student.Name}", "确认删除?", MessageBoxButtons.YesNo)== DialogResult.Yes)
{
students.Remove(student);
bindingSource.DataSource = GetPagedData(currentPage, pageSize);
MessageBox.Show($"删除成功: {student.Name}");
}
}
InputBox inputBox = new InputBox();
private void Btn_Add_Click(object sender, EventArgs e)
{
if (inputBox.OnValueComfirmChanged==null)
{
inputBox.OnValueComfirmChanged += ValueComfirmChangedCallback;
}
inputBox.Show();
}
private void ValueComfirmChangedCallback(object sender, object receiver)
{
if(receiver!=null && receiver is Student)
{
Student student = (Student)receiver;
student.ID = (10000 + students.Count).ToString();
students.Add(student);
ShowPageNumber();
}
inputBox.Hide();
}
public void ShowPageNumber()
{
int pageNum = 0;
if (students.Count % 10>0)
{
pageNum = students.Count / 10+1;
}
else
{
pageNum = students.Count / 10;
}
Label_CurrentPageShow.Text = $"{currentPage + 1}/{pageNum}";
}
}
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
private void InitializeComponent()
{
this.dataGridView1 = new System.Windows.Forms.DataGridView();
this.panel1 = new System.Windows.Forms.Panel();
this.Btn_Add = new System.Windows.Forms.Button();
this.panel2 = new System.Windows.Forms.Panel();
this.groupBox1 = new System.Windows.Forms.GroupBox();
this.panel3 = new System.Windows.Forms.Panel();
this.Btn_NextPage = new System.Windows.Forms.Button();
this.Btn_UpPage = new System.Windows.Forms.Button();
this.Label_CurrentPageShow = new System.Windows.Forms.Label();
((System.ComponentModel.ISupportInitialize)(this.dataGridView1)).BeginInit();
this.panel1.SuspendLayout();
this.panel2.SuspendLayout();
this.groupBox1.SuspendLayout();
this.panel3.SuspendLayout();
this.SuspendLayout();
this.dataGridView1.ColumnHeadersHeightSizeMode = System.Windows.Forms.DataGridViewColumnHeadersHeightSizeMode.AutoSize;
this.dataGridView1.Dock = System.Windows.Forms.DockStyle.Fill;
this.dataGridView1.Location = new System.Drawing.Point(3, 24);
this.dataGridView1.Name = "dataGridView1";
this.dataGridView1.RowHeadersWidth = 62;
this.dataGridView1.Size = new System.Drawing.Size(1079, 525);
this.dataGridView1.TabIndex = 0;
this.dataGridView1.CellContentClick += new System.Windows.Forms.DataGridViewCellEventHandler(this.dataGridView1_CellContentClick);
this.panel1.Controls.Add(this.Btn_Add);
this.panel1.Dock = System.Windows.Forms.DockStyle.Top;
this.panel1.Location = new System.Drawing.Point(0, 0);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(1085, 100);
this.panel1.TabIndex = 1;
this.Btn_Add.Location = new System.Drawing.Point(939, 48);
this.Btn_Add.Name = "Btn_Add";
this.Btn_Add.Size = new System.Drawing.Size(134, 46);
this.Btn_Add.TabIndex = 2;
this.Btn_Add.Text = "添加";
this.Btn_Add.UseVisualStyleBackColor = true;
this.Btn_Add.Click += new System.EventHandler(this.Btn_Add_Click);
this.panel2.Controls.Add(this.groupBox1);
this.panel2.Controls.Add(this.panel3);
this.panel2.Dock = System.Windows.Forms.DockStyle.Fill;
this.panel2.Location = new System.Drawing.Point(0, 100);
this.panel2.Name = "panel2";
this.panel2.Size = new System.Drawing.Size(1085, 652);
this.panel2.TabIndex = 2;
this.groupBox1.Controls.Add(this.dataGridView1);
this.groupBox1.Dock = System.Windows.Forms.DockStyle.Fill;
this.groupBox1.Location = new System.Drawing.Point(0, 0);
this.groupBox1.Name = "groupBox1";
this.groupBox1.Size = new System.Drawing.Size(1085, 552);
this.groupBox1.TabIndex = 1;
this.groupBox1.TabStop = false;
this.groupBox1.Text = "groupBox1";
this.panel3.Controls.Add(this.Label_CurrentPageShow);
this.panel3.Controls.Add(this.Btn_NextPage);
this.panel3.Controls.Add(this.Btn_UpPage);
this.panel3.Dock = System.Windows.Forms.DockStyle.Bottom;
this.panel3.Location = new System.Drawing.Point(0, 552);
this.panel3.Name = "panel3";
this.panel3.Size = new System.Drawing.Size(1085, 100);
this.panel3.TabIndex = 2;
this.Btn_NextPage.Location = new System.Drawing.Point(626, 42);
this.Btn_NextPage.Name = "Btn_NextPage";
this.Btn_NextPage.Size = new System.Drawing.Size(134, 46);
this.Btn_NextPage.TabIndex = 1;
this.Btn_NextPage.Text = "下一页";
this.Btn_NextPage.UseVisualStyleBackColor = true;
this.Btn_NextPage.Click += new System.EventHandler(this.btnNext_Click);
this.Btn_UpPage.Location = new System.Drawing.Point(370, 42);
this.Btn_UpPage.Name = "Btn_UpPage";
this.Btn_UpPage.Size = new System.Drawing.Size(134, 46);
this.Btn_UpPage.TabIndex = 0;
this.Btn_UpPage.Text = "上一页";
this.Btn_UpPage.UseVisualStyleBackColor = true;
this.Btn_UpPage.Click += new System.EventHandler(this.btnPrevious_Click);
this.Label_CurrentPageShow.AutoSize = true;
this.Label_CurrentPageShow.Font = new System.Drawing.Font("宋体", 12F);
this.Label_CurrentPageShow.Location = new System.Drawing.Point(530, 54);
this.Label_CurrentPageShow.Name = "Label_CurrentPageShow";
this.Label_CurrentPageShow.Size = new System.Drawing.Size(46, 24);
this.Label_CurrentPageShow.TabIndex = 2;
this.Label_CurrentPageShow.Text = "0/0";
this.AutoScaleDimensions = new System.Drawing.SizeF(9F, 18F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(1085, 752);
this.Controls.Add(this.panel2);
this.Controls.Add(this.panel1);
this.Name = "Form1";
this.Text = "Form1";
this.Load += new System.EventHandler(this.Form1_Load);
((System.ComponentModel.ISupportInitialize)(this.dataGridView1)).EndInit();
this.panel1.ResumeLayout(false);
this.panel2.ResumeLayout(false);
this.groupBox1.ResumeLayout(false);
this.panel3.ResumeLayout(false);
this.panel3.PerformLayout();
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.DataGridView dataGridView1;
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.Panel panel2;
private System.Windows.Forms.GroupBox groupBox1;
private System.Windows.Forms.Panel panel3;
private System.Windows.Forms.Button Btn_UpPage;
private System.Windows.Forms.Button Btn_NextPage;
private System.Windows.Forms.Button Btn_Add;
private System.Windows.Forms.Label Label_CurrentPageShow;
}
2、数据代码
Student
public class Student
{
public string ID { get; set; }
public string Name { get; set; }
public string Major { get; set; }
public Student()
{
}
public Student(string name ,string major)
{
Name = name;
Major = major;
}
}
3、输入框代码
public delegate void ValueComfirmChanged(object sender ,object receiver);
public ValueComfirmChanged OnValueComfirmChanged;
public InputBox()
{
InitializeComponent();
this.CenterToParent();
}
private void Btn_Comfirm_Click(object sender, EventArgs e)
{
OnValueComfirmChanged?.Invoke(this, new Student(Tbx_Name.Text,Tbx_Major.Text));
}
private void Btn_Cancel_Click(object sender, EventArgs e)
{
Tbx_Name.Clear();
Tbx_Major.Clear();
this.Hide();
}
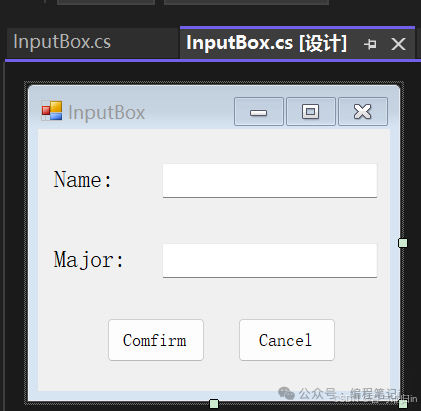
输入框设计器代码
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.Tbx_Name = new System.Windows.Forms.TextBox();
this.Tbx_Major = new System.Windows.Forms.TextBox();
this.label2 = new System.Windows.Forms.Label();
this.Btn_Comfirm = new System.Windows.Forms.Button();
this.Btn_Cancel = new System.Windows.Forms.Button();
this.SuspendLayout();
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("宋体", 12F);
this.label1.Location = new System.Drawing.Point(12, 37);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(70, 24);
this.label1.TabIndex = 0;
this.label1.Text = "Name:";
this.Tbx_Name.Font = new System.Drawing.Font("宋体", 12F);
this.Tbx_Name.Location = new System.Drawing.Point(124, 34);
this.Tbx_Name.Name = "Tbx_Name";
this.Tbx_Name.Size = new System.Drawing.Size(216, 35);
this.Tbx_Name.TabIndex = 1;
this.Tbx_Major.Font = new System.Drawing.Font("宋体", 12F);
this.Tbx_Major.Location = new System.Drawing.Point(124, 114);
this.Tbx_Major.Name = "Tbx_Major";
this.Tbx_Major.Size = new System.Drawing.Size(216, 35);
this.Tbx_Major.TabIndex = 3;
this.label2.AutoSize = true;
this.label2.Font = new System.Drawing.Font("宋体", 12F);
this.label2.Location = new System.Drawing.Point(12, 117);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(82, 24);
this.label2.TabIndex = 2;
this.label2.Text = "Major:";
this.Btn_Comfirm.Location = new System.Drawing.Point(69, 189);
this.Btn_Comfirm.Name = "Btn_Comfirm";
this.Btn_Comfirm.Size = new System.Drawing.Size(98, 44);
this.Btn_Comfirm.TabIndex = 4;
this.Btn_Comfirm.Text = "Comfirm";
this.Btn_Comfirm.UseVisualStyleBackColor = true;
this.Btn_Comfirm.Click += new System.EventHandler(this.Btn_Comfirm_Click);
this.Btn_Cancel.Location = new System.Drawing.Point(200, 189);
this.Btn_Cancel.Name = "Btn_Cancel";
this.Btn_Cancel.Size = new System.Drawing.Size(98, 44);
this.Btn_Cancel.TabIndex = 5;
this.Btn_Cancel.Text = "Cancel";
this.Btn_Cancel.UseVisualStyleBackColor = true;
this.Btn_Cancel.Click += new System.EventHandler(this.Btn_Cancel_Click);
this.AutoScaleDimensions = new System.Drawing.SizeF(9F, 18F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(352, 262);
this.Controls.Add(this.Btn_Cancel);
this.Controls.Add(this.Btn_Comfirm);
this.Controls.Add(this.Tbx_Major);
this.Controls.Add(this.label2);
this.Controls.Add(this.Tbx_Name);
this.Controls.Add(this.label1);
this.Name = "InputBox";
this.Text = "InputBox";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox Tbx_Name;
private System.Windows.Forms.TextBox Tbx_Major;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Button Btn_Comfirm;
private System.Windows.Forms.Button Btn_Cancel;
阅读原文:原文链接
该文章在 2025/3/31 11:18:22 编辑过